As a result, instead of executing /etc/init as it expects, the kernel's icode() actually executes something entirely different. I took the opportunity to test the argument- and environment-passing mechanism of execve() inside the new user program simply by printing the arguments and environment passed to it. That program essentially consists of this code:
#include <stdio.h>which is a fully-working standalone C program. You can run the above program and see all of the arguments and environment passed to it. Here is the result of running this program in Punix (the text after the copyright and warranty information but before the kernel panic message):
#include <string.h>
static void println(char *s)
{
write(2, s, strlen(s));
write(2, "\n", 1);
}
int main(int argc, char *argv[], char *envp[])
{
println("This is a user program.");
println("\narguments:");
for (; *argv; ++argv)
println(*argv);
println("\nenvironment:");
for (; *envp; ++envp)
println(*envp);
return 0;
}
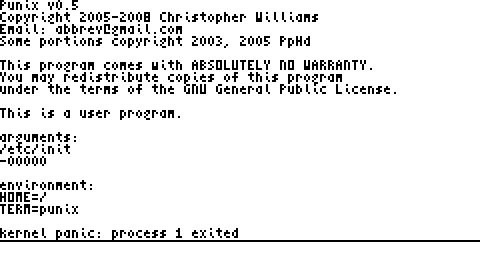
The arguments and environment are those passed to it by icode(). The first argument to the program, "-00000", comes from 2.11BSD (one of many sources I'm using to write Punix). I kept it in there when I transliterated my icode() routine from PDP to 68k assembly language code. When I get a real "init" program I'll change the argument to whatever it expects and accepts as arguments (such as to specify the run level to enter after booting). It will also depend on the (possible) presence of a boot loader that can pass arguments to the kernel and to init. That will be some time in the future, so I won't worry about it for now.